Text¶
Rationale¶
The text
module is used to create text output. It seamlessly integrates
Donald E. Knuths famous TeX typesetting engine[1]. The module is a
high-level interface to an extensive stack of TeX and font related
functionality in PyX, whose details are way beyond this manual and completely
irrelevant for the typical PyX user. However, the basic concept should be
described briefly, as it provides important insights into essential properties
of the whole machinery.
PyX does not apply any limitations on the text submitted by the user. Instead the text is directly passed to TeX. This has the implication, that the text to be typeset should come from a trusted source or some special security measures should be applied (see Typesetting insecure text). PyX just adds a light and transparent wrapper using basic TeX functionality for later identification and output extraction. This procedure enables full access to all TeX features and makes PyX on the other hand dependent on the error handling provided by TeX. However, a detailed and immediate control of the TeX output allows PyX to report problems back to the user as they occur.
While we only talked about TeX so far (and will continue to do so in the rest of this section), it is important to note that the coupling is not limited to plain TeX. Currently, PyX can also use LaTeX for typesetting, and other TeX variants could be added in the future. What PyX really depends on is the ability of the typesetting program to generate DVI[2].
As soon as some text creation is requested or, even before that, a preamble
setting or macro definition is submitted, the TeX program is started as a
separate process. The input and output is bound to a SingleEngine
instance. Typically, the process will be kept alive and will be reused for all
future typesetting requests until the end of the PyX process. However, there
are certain situations when the TeX program needs to be shutdown early, which
are be described in detail in the TeX ipc mode section.
Whenever PyX sends some commands to the TeX interpreter, it adds an output
marker at the end, and waits for this output marker to be echoed in the TeX
output. All intermediate output is attributed to the commands just sent and
will be analysed for problems. This is done by texmessage
parsers.
Here, a problem could be logged to the PyX logger at warning level, thus
be reported to stderr
by default. This happens for over- or underful boxes
or font warnings emitted by TeX. For other unknown problems (i.e. output not
handled by any of the given texmessage
parsers), a
TexResultError
is raised, which creates a detailed error report
including the traceback, the commands submitted to TeX and the output returned
by TeX.
PyX wraps each text to be typeset in a TeX box and adds a shipout of this box to the TeX code before forwarding it to TeX. Thus a page in the DVI file is created containing just this output. Furthermore TeX is asked to output the box extent. By that PyX will immediately know the size of the text without referring to the DVI. This also allows faking the box size by TeX means, as you would expect it.
Once the actual output is requested, PyX reads the content of the DVI file, accessing the page related to the output in question. It then does all the necessary steps to transform the DVI content to the requested output format, like searching for virtual font files, font metrices, font mapping files, and PostScript Type1 fonts to be used in the final output. Here a present limitation has been mentioned: PyX presently can use PostScript Type1 fonts only to generate text output. While this is a serious limitation, all the default fonts in TeX are available in Type1 nowadays and current TeX installations are alreadily configured to use them by default.
TeX interface¶
- class text.SingleEngine(cmd, texenc='ascii', usefiles=[], texipc=config.getboolean('text', 'texipc', 0), copyinput=None, dvitype=False, errordetail=errordetail.default, texmessages_start=[], texmessages_end=[], texmessages_preamble=[], texmessages_run=[])¶
Base class for the TeX interface.
Note
This class cannot be used directly. It is the base class for all tex engines and provides most of the implementation. Still, to the end user the parameters except for cmd are important, as they are preserved in derived classes usually.
- Parameters:
cmd (list of str) – command and arguments to start the TeX interpreter
texenc (str) – encoding to use in the communication with the TeX interpreter
usefiles (list of str) – list of supplementary files to be copied to and from the temporary working directory (see Debugging for usage details)
texipc (bool) – TeX ipc mode flag.
copyinput (None or str or file) – filename or file to be used to store a copy of all the input passed to the TeX interpreter
dvitype (bool) – flag to turn on dvitype-like output
errordetail (
errordetail
) – verbosity of theTexResultError
texmessages_start (list of
texmessage
parsers) – additional message parsers at interpreter startuptexmessages_end (list of
texmessage
parsers) – additional message parsers at interpreter shutdowntexmessages_preamble (list of
texmessage
parsers) – additional message parsers for preamble outputtexmessages_run (list of
texmessage
parsers) – additional message parsers for typset output
- texmessages_start_default = [<function texmessage.start>]¶
default
texmessage
parsers at interpreter startup
- texmessages_end_default = [<function texmessage.end>, <function texmessage.font_warning>, <function texmessage.rerun_warning>, <function texmessage.nobbl_warning>]¶
default
texmessage
parsers at interpreter shutdown
- texmessages_preamble_default = [<function texmessage.load>]¶
default
texmessage
parsers for preamble output
- texmessages_run_default = [<function texmessage.font_warning>, <function texmessage.box_warning>, <function texmessage.package_warning>, <function texmessage.load_def>, <function texmessage.load_graphics>]¶
default
texmessage
parsers for typeset output
- preamble(expr, texmessages=[])¶
Execute a preamble.
- Parameters:
expr (str) – expression to be executed
texmessages (list of
texmessage
parsers) – additional message parsers
Preambles must not generate output, but are used to load files, perform settings, define macros, etc. In LaTeX mode, preambles are executed before
\begin{document}
. The method can be called multiple times, but only prior toSingleEngine.text()
andSingleEngine.text_pt()
.
- text_pt(x_pt, y_pt, expr, textattrs=[], texmessages=[], fontmap=None, singlecharmode=False)¶
Typeset text.
- Parameters:
x_pt (float) – x position in pts
y_pt (float) – y position in pts
expr (str or
MultiEngineText
) – text to be typesettextattrs (list of
textattr, :class:`trafo.trafo_pt
, andstyle.fillstyle
) – styles and attributes to be applied to the texttexmessages (list of
texmessage
parsers) – additional message parsersfontmap (None or fontmap) – force a fontmap to be used (instead of the default depending on the output format)
singlecharmode (bool) – position each character separately
- Returns:
text output insertable into a canvas.
- Return type:
- Raises:
TexDoneError
: when the TeX interpreter has been terminated already.
- class text.SingleTexEngine(cmd=config.getlist('text', 'tex', ['tex']), lfs='10pt', **kwargs)¶
Plain TeX interface.
This class adjusts the
SingleEngine
to use plain TeX.- Parameters:
cmd (list of str) – command and arguments to start the TeX interpreter
lfs (str or None) – resemble LaTeX font settings within plain TeX by loading a lfs-file
kwargs – additional arguments passed to
SingleEngine
An lfs-file is a file defining a set of font commands like
\normalsize
by font selection commands in plain TeX. Several of those files resembling standard settings of LaTeX are distributed along with PyX in thepyx/data/lfs
directory. This directory also contains a LaTeX file to create lfs files for different settings (LaTeX class, class options, and style files).
- class text.SingleLatexEngine(cmd=config.getlist('text', 'latex', ['latex']), docclass='article', docopt=None, pyxgraphics=True, texmessages_docclass=[], texmessages_begindoc=[], **kwargs)¶
LaTeX interface.
This class adjusts the
SingleEngine
to use LaTeX.- Parameters:
cmd (list of str) – command and arguments to start the TeX interpreter in LaTeX mode
docclass (str) – document class
docopt (str or None) – document loading options
pyxgraphics (bool) – activate graphics bundle support, see Using the graphics-bundle with LaTeX
texmessages_docclass (list of
texmessage
parsers) – additional message parsers at LaTeX class loadingtexmessages_begindoc (list of
texmessage
parsers) – additional message parsers at\begin{document}
kwargs – additional arguments passed to
SingleEngine
- texmessages_docclass_default = [<function texmessage.load>]¶
default
texmessage
parsers at LaTeX class loading
- texmessages_begindoc_default = [<function texmessage.load>, <function texmessage.no_aux>]¶
default
texmessage
parsers at\begin{document}
The SingleEngine
classes described above do not handle restarts of the
interpreter when the actuall DVI result is required and is not available via
the TeX ipc mode feature.
The MultiEngine
classes below are not derived from
SingleEngine
even though the provide the same functional interface
(MultiEngine.preamble()
, MultiEngine.text()
, and
MultiEngine.text_pt()
), but instead wrap a SingleEngine
instance, and provide an automatic (or manual by the MultiEngine.reset()
function) restart of the interpreter as required.
- class text.MultiEngine(cls, *args, **kwargs)¶
A restartable
SingleEngine
class- Parameters:
cls (
SingleEngine
class) – the class being wrappedargs (list) – args at class instantiation
kwargs (dict) – keyword args at at class instantiation
- preamble(expr, texmessages=[])¶
resembles
SingleEngine.preamble()
- text_pt(*args, **kwargs)¶
resembles
SingleEngine.text_pt()
- text(*args, **kwargs)¶
resembles
SingleEngine.text()
- reset(reinit=False)¶
Start a new
SingleEngine
instance- Parameters:
reinit (bool) – replay
preamble()
calls on the new instance
After executing this function further preamble calls are allowed, whereas once a text output has been created,
preamble()
calls are forbidden.
- class text.TexEngine(*args, **kwargs)¶
A restartable
SingleTexEngine
class- Parameters:
args (list) – args at class instantiation
kwargs (dict) – keyword args at at class instantiation
- class text.LatexEngine(*args, **kwargs)¶
A restartable
SingleLatexEngine
class- Parameters:
args (list) – args at class instantiation
kwargs (dict) – keyword args at at class instantiation
- class text.textextbox_pt(x_pt, y_pt, left_pt, right_pt, height_pt, depth_pt, do_finish, fontmap, singlecharmode, fillstyles)¶
Text output.
An instance of this class contains the text output generated by PyX. It is a
baseclasses.canvasitem
and thus can be inserted into a canvas.- left¶
left extent of the text (PyX length)
- right¶
right extent of the text (PyX length)
- width¶
width of the text (PyX length)
- height¶
height of the text (PyX length)
- depth¶
height of the text (PyX length)
- marker(name)¶
Return the position of a marker.
- Parameters:
name (str) – name of the marker
- Returns:
position of the marker
- Return type:
tuple of two PyX lengths
This method returns the position of the marker of the given name within, previously defined by the
\PyXMarker{name}
macro in the typeset text. Please do not use the@
character within your name to prevent name clashes with PyX internal uses (although we don’t the marker feature internally right now).Similar to generating actual output, the marker function accesses the DVI output, stopping. The TeX ipc mode feature will allow for this access without stopping the TeX interpreter.
Module level functionality¶
The text module provides the public interface of the SingleEngine
class by module level functions. For that, a module level MultiEngine
is created and configured by the set()
function. Each time the
set()
function is called, the existing module level MultiEngine
is replaced by a new one.
- text.defaulttextengine¶
the current
MultiEngine
instance for the module level functions
- text.preamble¶
defaulttextengine.preamble (bound method)
- text.text_pt¶
defaulttextengine.text_pt (bound method)
- text.text¶
defaulttextengine.text (bound method)
- text.reset¶
defaulttextengine.reset (bound method)
- text.set(engine=None, cls=None, mode=None, *args, **kwargs)¶
Setup a new module level
MultiEngine
- Parameters:
engine – the module level engine object to be used, i.e.
TexEngine
,LatexEngine
, orUnicodeEngine
cls (Engine object, not instance) –
identical to engine
- deprecated:
use the engine argument instead
mode (str or None) –
"tex"
forTexEngine
or"latex"
forLatexEngine
with arbitraty capitalization- deprecated:
use the engine argument instead
args (list) – args at class instantiation
kwargs (dict) – keyword args at at class instantiation
- text.escapestring(s, replace={" ": "~", "$": r"\$", "&": r"\&", " "_": r"\_", "%": r"\%", "^": r"\string^", "~": r"\string~", "<": "{$<$}", ">": "{$>$}", "{": r"{$\{$}", "}": r"{$\}$}", "\\": r"{$\setminus$}", "|": r"{$\mid$}"})¶
Escapes ASCII characters such that they can be typeset by TeX/LaTeX
TeX output parsers¶
While running TeX (and variants thereof) a variety of information is written to
stdout
like status messages, details about file access, and also warnings
and errors. PyX reads all the output and analyses it. Some of the output is
triggered as a direct response to the TeX input and is thus easy to understand
for PyX. This includes page output information, but also workflow control
information injected by PyX into the input stream. PyX uses it to check the
communication and typeset progress. All the other output is handled by a list
of texmessage
parsers, an individual set of functions applied to the
TeX output one after the other. Each of the function receives the TeX output as
a string and return it back (maybe altered). Such a function must perform one
of the following actions in response to the TeX output is receives:
If it does not find any text in the TeX output it feals responsible for, it should just return the unchanged string.
If it finds a text it is responsible for, and the message is just fine (doesn’t need to be communicated to the user), it should just remove this text and return the rest of the TeX output.
If the text should be communicated to the user, a message should be written the the pyx logger at warning level, thus being reported to the user on
stderr
by default. Examples are underfull and overfull box warnings or font warnings. In addition, the text should be removed as in 2 above.In case of an error,
TexResultError
should be raised.
This is rather uncommon, that the fourth option is taken directly. Instead,
errors can just be kept in the output as PyX considers unhandled TeX output
left after applying all given texmessage
parsers as an error. In
addition to the error message, information about the TeX in- and output will be
added to the exception description text by the SingleEngine
according
to the errordetail
setting. The following verbosity levels are
available:
- class text.errordetail¶
Constants defining the verbosity of the
TexResultError
.- none = 0¶
Without any input and output.
- default = 1¶
Input and parsed output shortend to 5 lines.
- full = 2¶
Full input and unparsed as well as parsed output.
- exception text.TexResultError¶
Error raised by
texmessage
parsers.
To prevent any unhandled TeX output to be reported as an error,
texmessage.warn
or texmessage.ignore
can be used. To complete
the description, here is a list of all available texmessage
parsers:
- class text.texmessage¶
Collection of TeX output parsers.
This class is not meant to be instanciated. Instead, it serves as a namespace for TeX output parsers, which are functions receiving a TeX output and returning parsed output.
In addition, this class also contains some generator functions (namely
texmessage.no_file
andtexmessage.pattern
), which return a function according to the given parameters. They are used to generate some of the parsers in this class and can be used to create others as well.- static start(msg)¶
Validate TeX/LaTeX startup message including scrollmode test.
- Example:
>>> texmessage.start(r''' ... This is e-TeX (version) ... *! Undefined control sequence. ... <*> \raiseerror ... % ... ''') ''
- static no_file(fileending, qualname=None)¶
Generator function to ignore the missing file message for fileending.
- static no_aux(msg)¶
Ignore the missing aux file message.
Ignore the missing nav file message.
- static end(msg)¶
Validate TeX shutdown message.
- static load(msg)¶
Ignore file loading messages.
Removes text starting with a round bracket followed by a filename ignoring all further text until the corresponding closing bracket. Quotes and/or line breaks in the filename are handled as needed for TeX output.
Without quoting the filename, the necessary removal of line breaks is not well defined and the different possibilities are tested to check whether one solution is ok. The last of the examples below checks this behavior.
- Examples:
>>> texmessage.load(r'''other (text.py) things''') 'other things' >>> texmessage.load(r'''other ("text.py") things''') 'other things' >>> texmessage.load(r'''other ("tex ... t.py" further (ignored) ... text) things''') 'other things' >>> texmessage.load(r'''other (t ... ext ... .py ... fur ... ther (ignored) text) things''') 'other things'
- static load_def(msg)¶
Ignore font definition (
*.fd
and*.def
) loading messages.
- static load_graphics(msg)¶
Ignore graphics file (
*.eps
) loading messages.
- static ignore(msg)¶
Ignore all messages.
Should be used as a last resort only. You should write a proper TeX output parser function for the output you observe.
- static warn(msg)¶
Warn about all messages.
Similar to
ignore
, but writing a warning to the logger about the TeX output. This is considered to be better when you need to get it working quickly as you will still be prompted about the unresolved output, while the processing continues.
- static pattern(p, warning, qualname=None)¶
Warn by regular expression pattern matching.
- static box_warning(msg)¶
Warn about overfull/underfull box.
- static font_warning(msg)¶
Warn about font substitutions of NFSS.
- static package_warning(msg)¶
Warn about generic package messages.
- static rerun_warning(msg)¶
Warn about rerun required message.
- static nobbl_warning(msg)¶
Warn about no-bbl message.
TeX/LaTeX attributes¶
TeX/LaTeX attributes are instances to be passed to a texrunner
s
text()
method. They stand for TeX/LaTeX expression fragments and handle
dependencies by proper ordering.
- class text.halign(boxhalign, flushhalign)¶
Instances of this class set the horizontal alignment of a text box and the contents of a text box to be left, center and right for boxhalign and flushhalign being
0
,0.5
, and1
. Other values are allowed as well, although such an alignment seems quite unusual.
Note that there are two separate classes boxhalign
and
flushhalign
to set the alignment of the box and its contents
independently, but those helper classes can’t be cleared independently from each
other. Some handy instances available as class members:
- halign.boxleft¶
Left alignment of the text box, i.e. sets boxhalign to
0
and doesn’t set flushhalign.
- halign.boxcenter¶
Center alignment of the text box, i.e. sets boxhalign to
0.5
and doesn’t set flushhalign.
- halign.boxright¶
Right alignment of the text box, i.e. sets boxhalign to
1
and doesn’t set flushhalign.
- halign.flushleft¶
Left alignment of the content of the text box in a multiline box, i.e. sets flushhalign to
0
and doesn’t set boxhalign.
- halign.flushcenter¶
Center alignment of the content of the text box in a multiline box, i.e. sets flushhalign to
0.5
and doesn’t set boxhalign.
- halign.raggedcenter¶
Identical to
flushcenter
.
- halign.flushright¶
Right alignment of the content of the text box in a multiline box, i.e. sets flushhalign to
1
and doesn’t set boxhalign.
- halign.raggedleft¶
Identical to
flushright
.
- halign.center¶
Combines
boxcenter
andflushcenter
, i.e.halign(0.5, 0.5)
.
- halign.right¶
Combines
boxright
andflushright
, i.e.halign(1, 1)
.
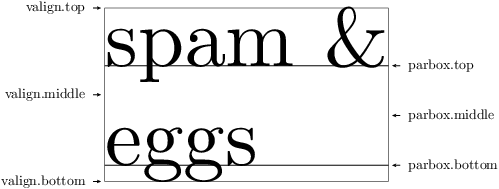
valign example¶
- class text.valign(valign)¶
Instances of this class set the vertical alignment of a text box to be top, center and bottom for valign being
0
,0.5
, and1
. Other values are allowed as well, although such an alignment seems quite unusual. See the left side of figure valign example for an example.
Some handy instances available as class members:
- valign.top¶
valign(0)
- valign.middle¶
valign(0.5)
- valign.bottom¶
valign(1)
- valign.baseline¶
Identical to clearing the vertical alignment by
clear
to emphasise that a baseline alignment is not a box-related alignment. Baseline alignment is the default, i.e. no valign is set by default.
- class text.parbox(width, baseline=top)¶
Instances of this class create a box with a finite width, where the typesetter creates multiple lines in. Note, that you can’t create multiple lines in TeX/LaTeX without specifying a box width. Since PyX doesn’t know a box width, it uses TeXs LR-mode by default, which will always put everything into a single line. Since in a vertical box there are several baselines, you can specify the baseline to be used by the optional baseline argument. You can set it to the symbolic names
top
,parbox.middle
, andparbox.bottom
only, which are members ofvalign
. See the right side of figure valign example for an example.
Since you need to specify a box width no predefined instances are available as class members.
- class text.vshift(lowerratio, heightstr='0')¶
Instances of this class lower the output by lowerratio of the height of the string heigthstring. Note, that you can apply several shifts to sum up the shift result. However, there is still a
clear
class member to remove all vertical shifts.
Some handy instances available as class members:
- vshift.bottomzero¶
vshift(0)
(this doesn’t shift at all)
- vshift.middlezero¶
vshift(0.5)
- vshift.topzero¶
vshift(1)
- vshift.mathaxis¶
This is a special vertical shift to lower the output by the height of the mathematical axis. The mathematical axis is used by TeX for the vertical alignment in mathematical expressions and is often usefull for vertical alignment. The corresponding vertical shift is less than
middlezero
and usually fits the height of the minus sign. (It is the height of the minus sign in mathematical mode, since that’s that the mathematical axis is all about.)
There is a TeX/LaTeX attribute to switch to TeXs math mode. The appropriate
instances mathmode
and clearmathmode
(to clear the math mode attribute)
are available at module level.
- text.mathmode¶
Enables TeXs mathematical mode in display style.
The size
class creates TeX/LaTeX attributes for changing the font size.
- class text.size(sizeindex=None, sizename=None, sizelist=defaultsizelist)¶
LaTeX knows several commands to change the font size. The command names are stored in the sizelist, which defaults to
["normalsize", "large", "Large", "LARGE", "huge", "Huge", None, "tiny", "scriptsize", "footnotesize", "small"]
.You can either provide an index sizeindex to access an item in sizelist or set the command name by sizename.
Instances for the LaTeXs default size change commands are available as class members:
- size.tiny¶
size(-4)
- size.scriptsize¶
size(-3)
- size.footnotesize¶
size(-2)
- size.small¶
size(-1)
- size.normalsize¶
size(0)
- size.large¶
size(1)
- size.Large¶
size(2)
- size.LARGE¶
size(3)
- size.huge¶
size(4)
- size.Huge¶
size(5)
There is a TeX/LaTeX attribute to create empty text boxes with the size of the
material passed in. The appropriate instances phantom
and clearphantom
(to clear the phantom attribute) are available at module level.
- text.phantom¶
Skip the text in the box, but keep its size.
Using the graphics-bundle with LaTeX¶
The packages in the LaTeX graphics bundle (color.sty
, graphics.sty
,
graphicx.sty
, …) make extensive use of \\special
commands. PyX
defines a clean set of such commands to fit the needs of the LaTeX graphics
bundle. This is done via the pyx.def
driver file, which tells the graphics
bundle about the syntax of the \\special
commands as expected by PyX. You
can install the driver file pyx.def
into your LaTeX search path and add the
content of both files color.cfg
and graphics.cfg
to your personal
configuration files[3]. After you have installed the cfg
files, please
use the text
module with unset pyxgraphics
keyword argument which
will switch off a convenience hack for less experienced LaTeX users. You can
then import the LaTeX graphics bundle packages and related packages (e.g.
rotating
, …) with the option pyx
, e.g.
\\usepackage[pyx]{color,graphicx}
. Note that the option pyx
is only
available with unset pyxgraphics keyword argument and a properly installed
driver file. Otherwise, omit the specification of a driver when loading the
packages.
When you define colors in LaTeX via one of the color models gray
, cmyk
,
rgb
, RGB
, hsb
, then PyX will use the corresponding values (one to
four real numbers). In case you use any of the named
colors in LaTeX, PyX
will use the corresponding predefined color (see module color
and the color
table at the end of the manual). The additional LaTeX color model pyx
allows
to use a PyX color expression, such as color.cmyk(0,0,0,0)
directly in
LaTeX. It is passed to PyX.
When importing Encapsulated PostScript files (eps
files) PyX will rotate,
scale and clip your file like you expect it. Other graphic formats can not be
imported via the graphics package at the moment.
For reference purpose, the following specials can be handled by PyX at the moment:
PyX:color_begin (model) (spec)
starts a color.
(model)
is one ofgray
,cmyk
,rgb
,hsb
,texnamed
, orpyxcolor
.(spec)
depends on the model: a name or some numbersPyX:color_end
ends a color.
PyX:epsinclude file= llx= lly= urx= ury= width= height= clip=0/1
includes an Encapsulated PostScript file (
eps
files). The values ofllx
toury
are in the files’ coordinate system and specify the part of the graphics that should become the specifiedwidth
andheight
in the outcome. The graphics may be clipped. The last three parameters are optional.PyX:scale_begin (x) (y)
begins scaling from the current point.
PyX:scale_end
ends scaling.
PyX:rotate_begin (angle)
begins rotation around the current point.
PyX:rotate_end
ends rotation.
Configuration¶
While the PyX configuration technically has nothing to do with the text module, we mention it here as part of the text module since its main purpose is the configuration of various aspects related to the typesetting of text.
PyX comes with reasonable defaults which should work out of the box on most TeX
installations. The default values are defined in the PyX source code itself and
are repeated in the system-wide config file in INI file format located at
pyx/data/pyxrc
. This file also contains a description of each of the listed
config values and is read at PyX startup. Thus the system-wide configuration
can be adjusted by editing this file.
In addition, a user-specific configuration can be setup by a ~/.pyxrc
on
unix-like Systems (including OS X) or pyxrc
in the directory defined by the
environment variable APPDATA
on MS Windows. This user-specific
configuration will overwrite the system-wide settings.
Yet another configuration can be set by the environment variable PYXRC
. The
given file will is loaded on top of the configuration defined in the previous
steps.
TeX ipc mode¶
For output generation of typeset text and to calculate the positions of markers
(see textbox_pt.marker()
) the DVI output of the TeX interpreter must be
read. In contrast, the text extent (textbox_pt.left
,
textbox_pt.right
, textbox_pt.width
, textbox_pt.height
,
textbox_pt.depth
) is available without accessing the DVI output, as the
TeX interpreter is instructed by PyX to output it to stdout, which is read and
analysed at the typesetting step immediately.
Since TeX interpreters usually buffer the DVI output, the interpreter itself
needs to be terminated to get the DVI output. As MultiEngine
instances
can start a new interpreter when needed, this does not harm the functionality
and happens more or less unnoticable. Still it generates some penalty in terms
of execution speed, which can become huge for certain situations (alternation
between typesetting and marker access).
One of the effects of the texipc
option available in almost all present TeX
interpreters is to flush the DVI output after each page. As PyX reads the DVI
output linearily, it can successfully read all output whithout stopping the TeX
interpreter. It is suggested to enable the texipc
feature in the
system-wide configuration if available in the TeX interpreter being used.
Debugging¶
PyX provides various functionality to collect details about the typesetting
process. First off all, PyX reads the output generated by the TeX interpreter
while it processes the text provided by the user. If the given
texmessage
parsers do not validate this output, an
TexResultError
is raised immediately. The verbosity of this output can
be adjusted by the errordetail
setting of the SingleEngine
.
This might help in some cases to identify an error in the text passed for
typesetting, but for more complicated problems, other help is required.
One possibility is to output the actual code passed to the TeX interpreter. For
that you can pass a file name or a file handle to the copyinput
argument of
the SingleEngine
. You can then process the text by the TeX interpreter
yourself to reproduce the issue outside of PyX.
Similarily you can also save the log output from the TeX interpreter. For that
you need to pass a log file name (with the suffix .log
) in the usefiles
argument (which is a list of files) of the SingleEngine
. This list of
files are saved and restored in the temporary directory used by the TeX
interpreter. While originally it is meant to share, for example, a .aux
file between several runs (for which the temporary directory is different and
removed after each run), it can do the same for the .log
file (where the
restore feature is needless, but does not harm). PyX takes care of the proper
\jobname
, hence you can choose the filename arbitrarily with the exception
of the suffix, as the suffix is kept during the save and restore.
Still, all this might not help to fully understand the problem you’re facing.
For example there might be situations, where it is not clear which TeX
interpreter is actually used (when several executables are available and the
path setup within the Python interpreter differs from the one used on the
shell). In those situations it might help to enable some additional logging
output created by PyX. PyX uses the logging module from the standard library
and logs to a logger named "pyx"
. By default, various information about
executing external programms and locating files will not be echoed, as it is
written at info level, but PyX provides a simple convenience function to enable
the output of this logging level. Just call the pyxinfo()
function
defined on the PyX package before actually start using the package in your
Python program:
- pyx.pyxinfo()¶
Make PyX a little verbose (for information or debugging)
This function enables info level on the
"pyx"
logger. It also adds some general information about the Python interpreter, the PyX installation, and the PyX configuration to the logger.
Typesetting insecure text¶
When typesetting text it is passed to a TeX interpreter unchanged[4]. This
is a security problem if the text does not come from a trusted source. While
full access to all typesetting features is not considered a problem, you should
bear in mind that TeX code can be used to read data from any other file
accessible to the TeX process. To surely prevent this process from accessing
any other data unrelated to the TeX installation, you can setup a chroot
environment for the TeX interpreter and configure PyX to use it. This can be
achieved by setting the chroot
option and adjusting the TeX interpreter
call and the filelocator
configuration in the pyxrc
.
UnicodeEngine¶
- class text.UnicodeEngine(fontname='cmr10', size=10, font_metric_type=FontMetricType.afm)¶
- class text.Text(text, scale=1, shift=0)¶
Text for the UnicodeEngine with basic typesetting features
- Parameters:
text (str) – text to be typeset
scale (float) – scale
shift (float) – vertical shift in units of the text size (without the scale)
- class text.StackedText(texts, frac=False, align=0)¶
Stack text above each other for the UnicodeEngine
- Parameters:
texts (list) – texts to be typeset above each other
frac (bool) – add a fractional line (for two texts only)
align (float) – horizontal alignment of the text where 0 is left, 0.5 is centered, and 1 is right
Footnotes